Appreciating Anonymous Methods
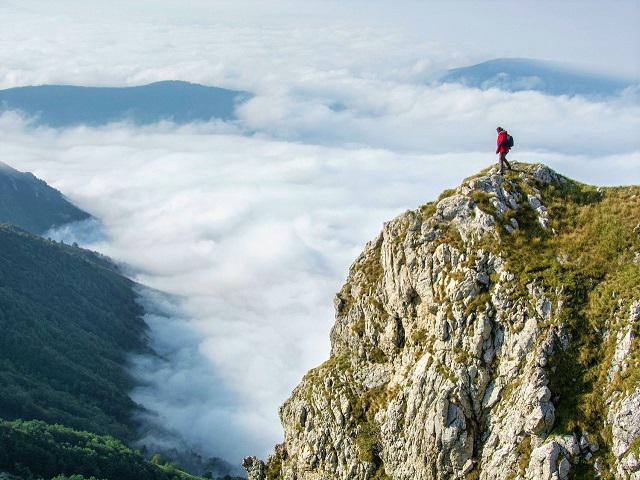
Anonymous methods can be hard to wrap your head around, but are an important step to understanding lambdas, now a mainstay of c# developments.
So what are anonymous methods?
Anonymous methods are, as the name might suggest, methods that do not have a name. Anonymous methods were introduced in c# 2.0 and mostly superseded by lambda expressions in c# 3.0. They are still valid, and important to understand.
Typically anonymous methods are passed to a method with a delegate parameter. They are purely Syntactic sugar to tidy up your code.
Let’s look at a concrete example.
We have an object that represents a calculation, with a method that accepts an operation to perform. The operation will be passed via a delegate. For example we pass a delegate to double the value, or square it, for example.
public delegate int operation (int value);
public class calculation {
private int _value;
public calculation(int initialValue) { _value = initialValue; }
public perform(operation op) { _value = op(_value); }
}
// matches operation delegate, so can be used by perform method
public int double(int value) {
return 2 * value;
}
// matches operation delegate, so can be used by perform method
public int square(int value) {
return value * value;
}
calculation example = new calculation(10);
example.perform(double); //example value is now 20
example.perform(square); //example value is now 400
Now let’s do the same thing, but get rid of our square method and use a anonymous method:
public delegate int operation (int value);
public class calculation {
private int _value;
public calculation(int initialValue) { _value = initialValue; }
public perform(operation op) { _value = op(_value); }
}
// matches operation delegate, so can be used by perform method
public int double(int value) {
return 2 * value;
}
calculation example = new calculation(10);
example.perform(double); //example value is now 20
example.perform(
delegate(int theValue) {
return theValue * theValue; }); //example value is now 400
Further
Anonymous methods can access outer variables, that is variables accessible in the block that declares the anonymous method.
public delegate int operation (int value);
public void example(int outerParam) {
string outerVar = "Bold";
operation op = delegate(int theValue) {
// Can access outerParam
// Can access outerVar
});
}
Considerations
- Jump statements, such as goto, break, or continue, inside an anonymous method are errors if their target is outside the block. Jump statements outside anonymous methods are also errors if their targets are inside an anonymous method.
- An anonymous method cannot access the in, ref or out parameters of an outer scope.
- No unsafe code can be accessed within the anonymous method.
- Anonymous methods are not allowed on the left side of the is operator.
Why Should I Care?
Well, as I said above, they are purely Syntactic sugar, to make your code easier to read. If a method is only used once, and it’s passed, as a delegate, to another method, then why clutter up your class with a method declaration?
Appreciating Anonymous Methods
Hopefully you can see how anonymous methods can be useful, their abilities and limitations, and that they are nothing to be afraid of! If you can think of situations where you might use anonymous methods then you might want to read up on lambda expressions.
Next Steps
Use the buttons below to follow the Bold Programmer on social media, so you don’t miss any future posts, and please leave a comment below if you found this article useful, confusing (or found a bug)